I had a .Net core 2.2 web API project which was created without the angular template. When we started the project, we did not plan to create any UI around these APIs but after a few releases, the needs changed and as an improvement, we decided to add client APP with angular to the same project.
Below are the steps to achieve this.
This article is not to show how angular CLI works or anything about angular but purely to see how to integrate angular app with .Net core 2.2 web API project.
Please make sure you have angular cli installed and have basic understanding of angular CLI and .Net core. Also, this is assuming that you have a web.api project created in .Net core.
Step 1: Lets go to the root folder and create a new client app using angular cli.
ng new ClientApp
This should create a basic client application with angular in ClientApp folder at the root level.
Add below line in Startup.cs file’s ConfigureServices method. This is basically telling .net core that static files will be served from ClientApp/dist folder which is the output folder for angular cli build. (Can be change in angular.json file)
app.UseSpa(spa =>
{
spa.Options.SourcePath = “ClientApp”;
if (env.IsDevelopment())
{
spa.UseProxyToSpaDevelopmentServer(“http://localhost:4200");
}
});
In the same file, add below lines in Configure method. This should enable .net core app to serve the static files
app.UseSpaStaticFiles();
For UI related development, we can use angular CLI from the ClientApp folder as any other angular application by running
ng serve -o
As you might already know, it starts the angular application on port 4200. Below line is to to make development life with .net core and angular easier. This is telling .Net that SPA application is running at port 4200 . Please associate yourself with that port. If there are any requests coming from this port to the server, those treat as internal to .net mvc routes
app.UseSpa(spa =>
{
spa.Options.SourcePath = “ClientApp”;
if (env.IsDevelopment())
{
spa.UseProxyToSpaDevelopmentServer(“http://localhost:4200");
}
});
Now if we run Net core app with F5 and angular app with “ng serve -o”, we see this
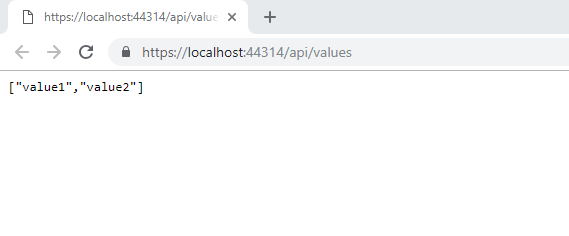
and if we remove /api/values, we get our angular app
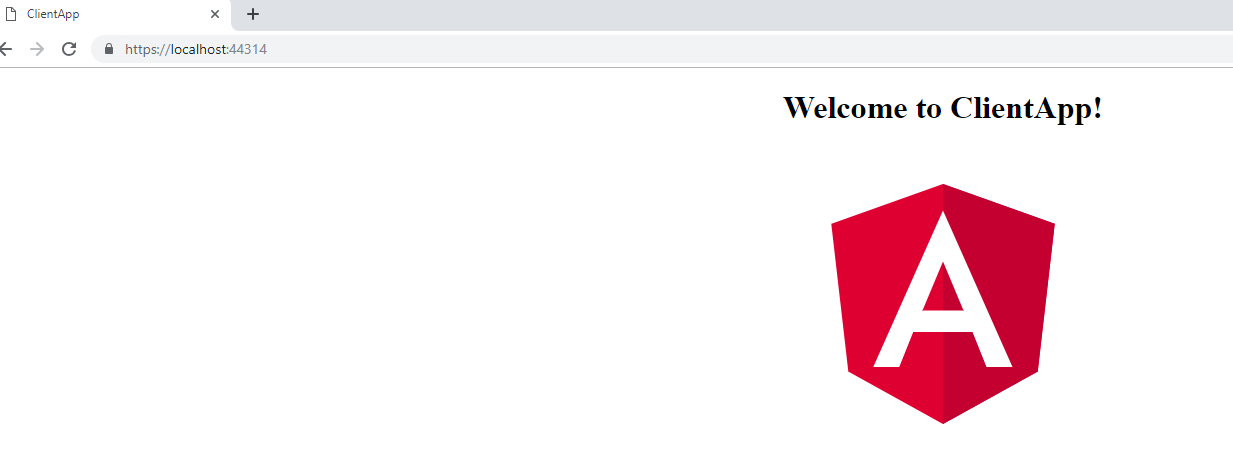
This should respond to all code changes in ts files as any other angular application and work seamlessly with .Net core APIs
Now this was for the development. To get the deployment/publish portion correct, edit project file of .Net core web api project and add below.
This portion is taken as is from the angular template of .Net core Web Application project.
<ItemGroup>
<! — Don’t publish the SPA source files, but do show them in the project files list →
<Content Remove=”$(SpaRoot)**” />
<None Include=”$(SpaRoot)**” Exclude=”$(SpaRoot)node_modules\**” />
</ItemGroup>
<Target Name=”DebugEnsureNodeEnv” BeforeTargets=”Build” Condition=” ‘$(Configuration)’ == ‘Debug’ And !Exists(‘$(SpaRoot)node_modules’) “>
<! — Ensure Node.js is installed →
<Exec Command=”node — version” ContinueOnError=”true”>
<Output TaskParameter=”ExitCode” PropertyName=”ErrorCode” />
</Exec>
<Message Importance=”high” Text=”Restoring dependencies using ‘npm’. This may take several minutes…” />
<Exec WorkingDirectory=”$(SpaRoot)” Command=”npm install” />
</Target>
<Target Name=”PublishRunWebpack” AfterTargets=”ComputeFilesToPublish”>
<! — As part of publishing, ensure the JS resources are freshly built in production mode →
<Exec WorkingDirectory=”$(SpaRoot)” Command=”npm install” />
<Exec WorkingDirectory=”$(SpaRoot)” Command=”npm run build — — prod” />
<Exec WorkingDirectory=”$(SpaRoot)” Command=”npm run build:ssr — — prod” Condition=” ‘$(BuildServerSideRenderer)’ == ‘true’ “ />
<! — Include the newly-built files in the publish output →
<ItemGroup>
<DistFiles Include=”$(SpaRoot)dist\**; $(SpaRoot)dist-server\**” />
<DistFiles Include=”$(SpaRoot)node_modules\**” Condition=”’$(BuildServerSideRenderer)’ == ‘true’” />
<ResolvedFileToPublish Include=”@(DistFiles->’%(FullPath)’)” Exclude=”@(ResolvedFileToPublish)”>
<RelativePath>%(DistFiles.Identity)</RelativePath>
<CopyToPublishDirectory>PreserveNewest</CopyToPublishDirectory>
</ResolvedFileToPublish>
</ItemGroup>
</Target>
Now, if you publish the web API application, it will extract the javascript files generated by angular cli into ClientApp/Dist folder. Also notice that none of the .ts files are published as those are not required.
Happy Coding!!